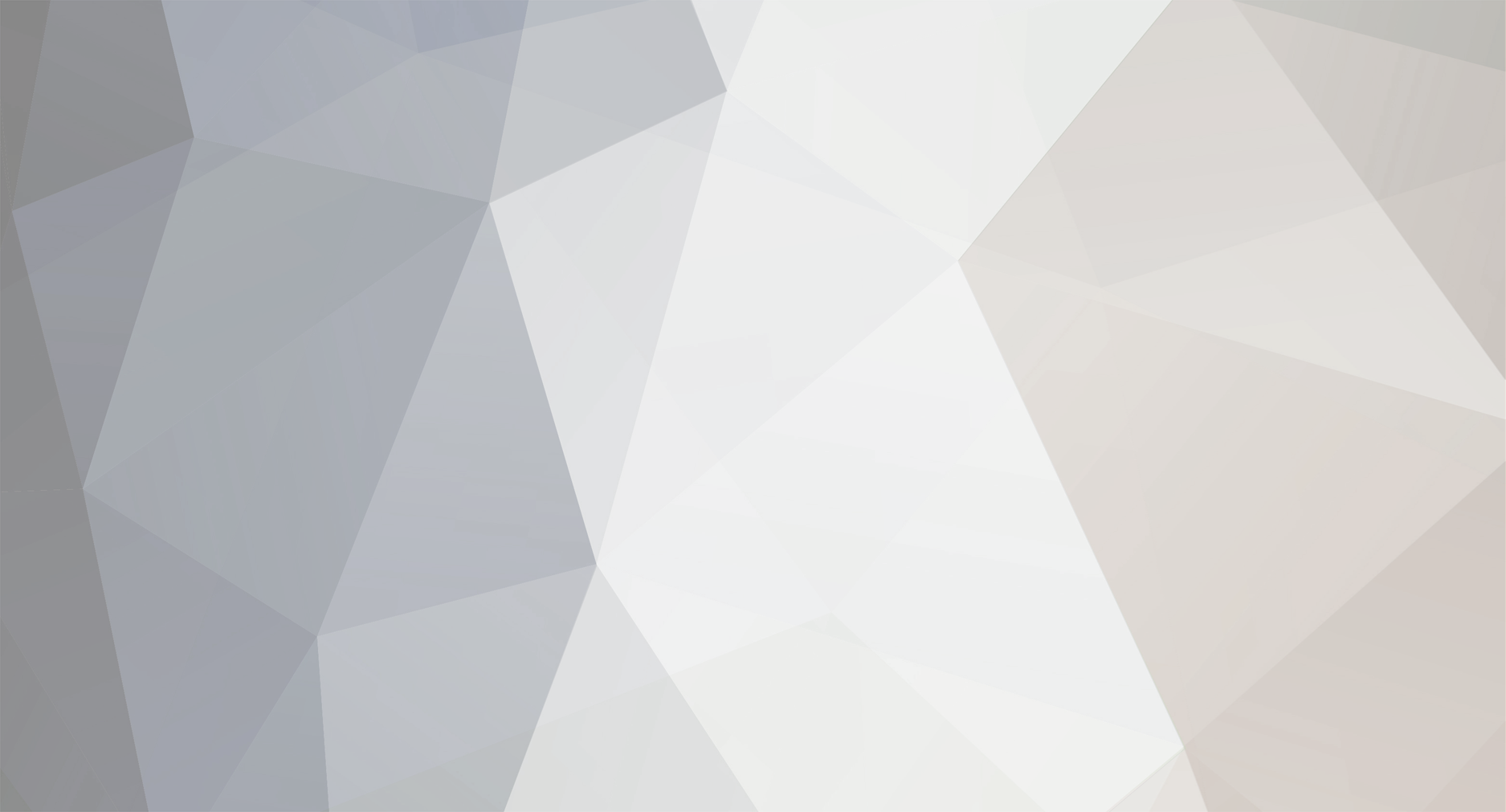
TI.ME
-
Posts
51 -
Joined
-
Last visited
-
Days Won
1
Content Type
Profiles
Forums
Events
Posts posted by TI.ME
-
-
On 9/18/2020 at 9:11 AM, ALFEU said:
paste an image in UniHTMLMemo I need to save it in bmp, jpeg, base64 or another format to save and send, if anyone has an example
Did you get a solution for this case?
-
Good Morning...
Why not use push directly on unigui without going through Onesignal or FireBase?-
1
-
-
3 hours ago, marcosdario2020 said:
Como assim?
Ele QR q vc arrume o seu cadastro preenchendo os dados da licença do unigui...
-
16 hours ago, TI.ME said:
Sorry, I was unable to attach the file, so I will leave the unit in writing ... This unit is where the export process is located, no installation or any other dependency is required ... to call the export just declare the unit and call the procedure ...
XLSWriter.DataSetToXLS(DataSet,Arq_Name);
Sorry, but I'm using a translator ... Report if it worked ... Good code ... Hugs
unit uNativeXLSExport;
// based on internet, generate basic BIFF5 XLS
// http://sc.openoffice.org/excelfileformat.pdf
// CodePage support (see WriteCodePage)
// and Unicode compatibility - Radek Cervinka, delphi.cz
interfaceuses
Windows, Messages, SysUtils, Classes, Graphics, Controls, Grids, Forms,
Dialogs, db, dbctrls, comctrls;const
{ BOF }
CBOF = $0009;
BIT_BIFF5 = $0800;
BOF_BIFF5 = CBOF or BIT_BIFF5;
{ EOF }
BIFF_EOF = $000A;
{ Document types }
DOCTYPE_XLS = $0010;
{ Dimensions }
DIMENSIONS = $0000;type
TAtributCell = (acHidden, acLocked, acShaded, acBottomBorder, acTopBorder,
acRightBorder, acLeftBorder, acLeft, acCenter, acRight, acFill);TSetOfAtribut = set of TAtributCell;
TXLSWriter = class(Tobject)
procedure DataSetToXLS(ds: TDataSet; fname: String);
private
maxCols, maxRows: word;
fstream: TFileStream;
procedure WriteWord(w: word);
procedure CellWord(vCol, vRow: word; aValue: word;
vAtribut: TSetOfAtribut = []);
procedure CellDouble(vCol, vRow: word; aValue: double;
vAtribut: TSetOfAtribut = []);
procedure CellStr(vCol, vRow: word; aValue: String;
vAtribut: TSetOfAtribut = []);
procedure WriteField(vCol, vRow: word; Field: TField);
constructor create(vFileName: string);
destructor Destroy; override;
procedure SetCellAtribut(value: TSetOfAtribut; var FAtribut: array of byte);
procedure StringGridToXLS(grid: TStringGrid; fname: String);protected
procedure WriteBOF;
procedure WriteEOF;
procedure WriteDimension;
procedure WriteCodePage;public
end;implementation
procedure TXLSWriter.DataSetToXLS(ds: TDataSet; fname: String);
var
c, r: Integer;
xls: TXLSWriter;
begin
xls := TXLSWriter.create(fname);
if ds.FieldCount > xls.maxCols then
xls.maxCols := ds.FieldCount + 1;
try
xls.WriteBOF;
xls.WriteCodePage;xls.WriteDimension;
for c := 0 to ds.FieldCount - 1 do
xls.CellStr(0, c, ds.Fields[c].FieldName);
r := 1;
ds.first;
while (not ds.eof) and (r <= xls.maxRows) do
begin
for c := 0 to ds.FieldCount - 1 do
xls.WriteField(r, c, ds.Fields[c]);
inc(r);
ds.next;
end;
xls.WriteEOF;// <2002-11-17> dllee
// ?? Dimension ?? wirteEOF ??,???? if ??? Seek ?? position
// if r > xls.maxrows then begin
// xls.maxrows:=r+1;
// xls.fstream.Seek(10,soFromBeginning);
// xls.WriteDimension;
// end;
// ????? maxrows ?????,????????? 65535,??,?????
finally
xls.free;
end;
end;procedure TXLSWriter.StringGridToXLS(grid: TStringGrid; fname: String);
var
c, r, rMax: Integer;
xls: TXLSWriter;
begin
xls := TXLSWriter.create(fname);
rMax := grid.RowCount;
if grid.ColCount > xls.maxCols then
xls.maxCols := grid.ColCount + 1;
if rMax > xls.maxRows then // ???????? 65535 Rows
rMax := xls.maxRows;
try
xls.WriteBOF;
xls.WriteDimension;
for c := 0 to grid.ColCount - 1 do
for r := 0 to rMax - 1 do
xls.CellStr(r, c, grid.Cells[c, r]);
xls.WriteEOF;
finally
xls.free;
end;
end;{ TXLSWriter }
constructor TXLSWriter.create(vFileName: string);
begin
inherited create;
if FileExists(vFileName) then
begin
fstream := TFileStream.create(vFileName, fmOpenWrite);
fstream.Size := 0;
end
else
fstream := TFileStream.create(vFileName, fmCreate);maxCols := 100; // <2002-11-17> dllee Column ???????? 65535, ??????
maxRows := 65535; // <2002-11-17> dllee ???????????,?????????????????
end;destructor TXLSWriter.destroy;
begin
if fstream <> nil then
fstream.free;
inherited;
end;procedure TXLSWriter.WriteBOF;
begin
WriteWord(BOF_BIFF5);
WriteWord(6); // count of bytes
WriteWord(0);
WriteWord(DOCTYPE_XLS);
WriteWord(0);
end;procedure TXLSWriter.WriteDimension;
begin
WriteWord(DIMENSIONS); // dimension OP Code
WriteWord(8); // count of bytes
WriteWord(0); // min cols
WriteWord(maxRows); // max rows
WriteWord(0); // min rowss
WriteWord(maxCols); // max cols
end;procedure TXLSWriter.CellDouble(vCol, vRow: word; aValue: double;
vAtribut: TSetOfAtribut);
var
FAtribut: array [0 .. 2] of byte;
begin
WriteWord(3); // opcode for double
WriteWord(15); // count of byte
WriteWord(vCol);
WriteWord(vRow);
SetCellAtribut(vAtribut, FAtribut);
fstream.Write(FAtribut, 3);
fstream.Write(aValue, 8);
end;procedure TXLSWriter.CellWord(vCol, vRow: word; aValue: word;
vAtribut: TSetOfAtribut = []);
var
FAtribut: array [0 .. 2] of byte;
begin
WriteWord(2); // opcode for word
WriteWord(9); // count of byte
WriteWord(vCol);
WriteWord(vRow);
SetCellAtribut(vAtribut, FAtribut);
fstream.Write(FAtribut, 3);
WriteWord(aValue);
end;procedure TXLSWriter.CellStr(vCol, vRow: word; aValue: String;
vAtribut: TSetOfAtribut);
var
FAtribut: array [0 .. 2] of byte;
slen: byte;
begin
WriteWord(4); // opcode for string
slen := length(aValue);
WriteWord(slen + 8); // count of byte
WriteWord(vCol);
WriteWord(vRow);SetCellAtribut(vAtribut, FAtribut);
fstream.Write(FAtribut, 3);fstream.Write(slen, 1);
{$IFDEF UNICODE}
fstream.Write(AnsiString(aValue)[1], slen);
{$ELSE}
fstream.Write(aValue[1], slen);
{$ENDIF}
end;procedure TXLSWriter.SetCellAtribut(value: TSetOfAtribut; var FAtribut: array of byte);
var
i: Integer;
begin
// reset
for i := 0 to High(FAtribut) do
FAtribut := 0;{ Byte Offset Bit Description Contents
0 7 Cell is not hidden 0b
Cell is hidden 1b
6 Cell is not locked 0b
Cell is locked 1b
5-0 Reserved, must be 0 000000b
1 7-6 Font number (4 possible)
5-0 Cell format code
2 7 Cell is not shaded 0b
Cell is shaded 1b
6 Cell has no bottom border 0b
Cell has a bottom border 1b
5 Cell has no top border 0b
Cell has a top border 1b
4 Cell has no right border 0b
Cell has a right border 1b
3 Cell has no left border 0b
Cell has a left border 1b
2-0 Cell alignment code
general 000b
left 001b
center 010b
right 011b
fill 100b
Multiplan default align. 111b
}// bit sequence 76543210
if acHidden in value then // byte 0 bit 7:
FAtribut[0] := FAtribut[0] + 128;if acLocked in value then // byte 0 bit 6:
FAtribut[0] := FAtribut[0] + 64;if acShaded in value then // byte 2 bit 7:
FAtribut[2] := FAtribut[2] + 128;if acBottomBorder in value then // byte 2 bit 6
FAtribut[2] := FAtribut[2] + 64;if acTopBorder in value then // byte 2 bit 5
FAtribut[2] := FAtribut[2] + 32;if acRightBorder in value then // byte 2 bit 4
FAtribut[2] := FAtribut[2] + 16;if acLeftBorder in value then // byte 2 bit 3
FAtribut[2] := FAtribut[2] + 8;// <2002-11-17> dllee ?? 3 bit ??? 1 ???
if acLeft in value then // byte 2 bit 1
FAtribut[2] := FAtribut[2] + 1
else if acCenter in value then // byte 2 bit 1
FAtribut[2] := FAtribut[2] + 2
else if acRight in value then // byte 2, bit 0 dan bit 1
FAtribut[2] := FAtribut[2] + 3
else if acFill in value then // byte 2, bit 0
FAtribut[2] := FAtribut[2] + 4;
end;procedure TXLSWriter.WriteWord(w: word);
begin
fstream.Write(w, 2);
end;procedure TXLSWriter.WriteEOF;
begin
WriteWord(BIFF_EOF);
WriteWord(0);
end;procedure TXLSWriter.WriteField(vCol, vRow: word; Field: TField);
begin
case Field.DataType of
ftString, ftWideString, ftBoolean, ftDate, ftDateTime, ftTime, ftWideMemo:
CellStr(vCol, vRow, Field.asstring);
ftAutoInc, ftSmallint, ftInteger, ftWord:
CellWord(vCol, vRow, Field.AsInteger);
ftFloat, ftBCD:
CellDouble(vCol, vRow, Field.AsFloat);
else
CellStr(vCol, vRow, EmptyStr); // <2002-11-17> dllee ??????????
end;
end;procedure TXLSWriter.WriteCodePage;
begin
WriteWord($0042); // OPCODE CODEPAGE
WriteWord($0002); // size
WriteWord($04E2); // CP1250
//- >http://sc.openoffice.org/excelfileformat.pdf , section 5.17
end;end.
Take a look at this code .. simple way to export to excel without dependencies ..
Att:
Gustavo Déo
-
4 hours ago, lfgarrido said:
Hello, could you share please?
Sorry, I was unable to attach the file, so I will leave the unit in writing ... This unit is where the export process is located, no installation or any other dependency is required ... to call the export just declare the unit and call the procedure ...
XLSWriter.DataSetToXLS(DataSet,Arq_Name);
Sorry, but I'm using a translator ... Report if it worked ... Good code ... Hugs
unit uNativeXLSExport;
// based on internet, generate basic BIFF5 XLS
// http://sc.openoffice.org/excelfileformat.pdf
// CodePage support (see WriteCodePage)
// and Unicode compatibility - Radek Cervinka, delphi.cz
interfaceuses
Windows, Messages, SysUtils, Classes, Graphics, Controls, Grids, Forms,
Dialogs, db, dbctrls, comctrls;const
{ BOF }
CBOF = $0009;
BIT_BIFF5 = $0800;
BOF_BIFF5 = CBOF or BIT_BIFF5;
{ EOF }
BIFF_EOF = $000A;
{ Document types }
DOCTYPE_XLS = $0010;
{ Dimensions }
DIMENSIONS = $0000;type
TAtributCell = (acHidden, acLocked, acShaded, acBottomBorder, acTopBorder,
acRightBorder, acLeftBorder, acLeft, acCenter, acRight, acFill);TSetOfAtribut = set of TAtributCell;
TXLSWriter = class(Tobject)
procedure DataSetToXLS(ds: TDataSet; fname: String);
private
maxCols, maxRows: word;
fstream: TFileStream;
procedure WriteWord(w: word);
procedure CellWord(vCol, vRow: word; aValue: word;
vAtribut: TSetOfAtribut = []);
procedure CellDouble(vCol, vRow: word; aValue: double;
vAtribut: TSetOfAtribut = []);
procedure CellStr(vCol, vRow: word; aValue: String;
vAtribut: TSetOfAtribut = []);
procedure WriteField(vCol, vRow: word; Field: TField);
constructor create(vFileName: string);
destructor Destroy; override;
procedure SetCellAtribut(value: TSetOfAtribut; var FAtribut: array of byte);
procedure StringGridToXLS(grid: TStringGrid; fname: String);protected
procedure WriteBOF;
procedure WriteEOF;
procedure WriteDimension;
procedure WriteCodePage;public
end;implementation
procedure TXLSWriter.DataSetToXLS(ds: TDataSet; fname: String);
var
c, r: Integer;
xls: TXLSWriter;
begin
xls := TXLSWriter.create(fname);
if ds.FieldCount > xls.maxCols then
xls.maxCols := ds.FieldCount + 1;
try
xls.WriteBOF;
xls.WriteCodePage;xls.WriteDimension;
for c := 0 to ds.FieldCount - 1 do
xls.CellStr(0, c, ds.Fields[c].FieldName);
r := 1;
ds.first;
while (not ds.eof) and (r <= xls.maxRows) do
begin
for c := 0 to ds.FieldCount - 1 do
xls.WriteField(r, c, ds.Fields[c]);
inc(r);
ds.next;
end;
xls.WriteEOF;// <2002-11-17> dllee
// ?? Dimension ?? wirteEOF ??,???? if ??? Seek ?? position
// if r > xls.maxrows then begin
// xls.maxrows:=r+1;
// xls.fstream.Seek(10,soFromBeginning);
// xls.WriteDimension;
// end;
// ????? maxrows ?????,????????? 65535,??,?????
finally
xls.free;
end;
end;procedure TXLSWriter.StringGridToXLS(grid: TStringGrid; fname: String);
var
c, r, rMax: Integer;
xls: TXLSWriter;
begin
xls := TXLSWriter.create(fname);
rMax := grid.RowCount;
if grid.ColCount > xls.maxCols then
xls.maxCols := grid.ColCount + 1;
if rMax > xls.maxRows then // ???????? 65535 Rows
rMax := xls.maxRows;
try
xls.WriteBOF;
xls.WriteDimension;
for c := 0 to grid.ColCount - 1 do
for r := 0 to rMax - 1 do
xls.CellStr(r, c, grid.Cells[c, r]);
xls.WriteEOF;
finally
xls.free;
end;
end;{ TXLSWriter }
constructor TXLSWriter.create(vFileName: string);
begin
inherited create;
if FileExists(vFileName) then
begin
fstream := TFileStream.create(vFileName, fmOpenWrite);
fstream.Size := 0;
end
else
fstream := TFileStream.create(vFileName, fmCreate);maxCols := 100; // <2002-11-17> dllee Column ???????? 65535, ??????
maxRows := 65535; // <2002-11-17> dllee ???????????,?????????????????
end;destructor TXLSWriter.destroy;
begin
if fstream <> nil then
fstream.free;
inherited;
end;procedure TXLSWriter.WriteBOF;
begin
WriteWord(BOF_BIFF5);
WriteWord(6); // count of bytes
WriteWord(0);
WriteWord(DOCTYPE_XLS);
WriteWord(0);
end;procedure TXLSWriter.WriteDimension;
begin
WriteWord(DIMENSIONS); // dimension OP Code
WriteWord(8); // count of bytes
WriteWord(0); // min cols
WriteWord(maxRows); // max rows
WriteWord(0); // min rowss
WriteWord(maxCols); // max cols
end;procedure TXLSWriter.CellDouble(vCol, vRow: word; aValue: double;
vAtribut: TSetOfAtribut);
var
FAtribut: array [0 .. 2] of byte;
begin
WriteWord(3); // opcode for double
WriteWord(15); // count of byte
WriteWord(vCol);
WriteWord(vRow);
SetCellAtribut(vAtribut, FAtribut);
fstream.Write(FAtribut, 3);
fstream.Write(aValue, 8);
end;procedure TXLSWriter.CellWord(vCol, vRow: word; aValue: word;
vAtribut: TSetOfAtribut = []);
var
FAtribut: array [0 .. 2] of byte;
begin
WriteWord(2); // opcode for word
WriteWord(9); // count of byte
WriteWord(vCol);
WriteWord(vRow);
SetCellAtribut(vAtribut, FAtribut);
fstream.Write(FAtribut, 3);
WriteWord(aValue);
end;procedure TXLSWriter.CellStr(vCol, vRow: word; aValue: String;
vAtribut: TSetOfAtribut);
var
FAtribut: array [0 .. 2] of byte;
slen: byte;
begin
WriteWord(4); // opcode for string
slen := length(aValue);
WriteWord(slen + 8); // count of byte
WriteWord(vCol);
WriteWord(vRow);SetCellAtribut(vAtribut, FAtribut);
fstream.Write(FAtribut, 3);fstream.Write(slen, 1);
{$IFDEF UNICODE}
fstream.Write(AnsiString(aValue)[1], slen);
{$ELSE}
fstream.Write(aValue[1], slen);
{$ENDIF}
end;procedure TXLSWriter.SetCellAtribut(value: TSetOfAtribut; var FAtribut: array of byte);
var
i: Integer;
begin
// reset
for i := 0 to High(FAtribut) do
FAtribut := 0;{ Byte Offset Bit Description Contents
0 7 Cell is not hidden 0b
Cell is hidden 1b
6 Cell is not locked 0b
Cell is locked 1b
5-0 Reserved, must be 0 000000b
1 7-6 Font number (4 possible)
5-0 Cell format code
2 7 Cell is not shaded 0b
Cell is shaded 1b
6 Cell has no bottom border 0b
Cell has a bottom border 1b
5 Cell has no top border 0b
Cell has a top border 1b
4 Cell has no right border 0b
Cell has a right border 1b
3 Cell has no left border 0b
Cell has a left border 1b
2-0 Cell alignment code
general 000b
left 001b
center 010b
right 011b
fill 100b
Multiplan default align. 111b
}// bit sequence 76543210
if acHidden in value then // byte 0 bit 7:
FAtribut[0] := FAtribut[0] + 128;if acLocked in value then // byte 0 bit 6:
FAtribut[0] := FAtribut[0] + 64;if acShaded in value then // byte 2 bit 7:
FAtribut[2] := FAtribut[2] + 128;if acBottomBorder in value then // byte 2 bit 6
FAtribut[2] := FAtribut[2] + 64;if acTopBorder in value then // byte 2 bit 5
FAtribut[2] := FAtribut[2] + 32;if acRightBorder in value then // byte 2 bit 4
FAtribut[2] := FAtribut[2] + 16;if acLeftBorder in value then // byte 2 bit 3
FAtribut[2] := FAtribut[2] + 8;// <2002-11-17> dllee ?? 3 bit ??? 1 ???
if acLeft in value then // byte 2 bit 1
FAtribut[2] := FAtribut[2] + 1
else if acCenter in value then // byte 2 bit 1
FAtribut[2] := FAtribut[2] + 2
else if acRight in value then // byte 2, bit 0 dan bit 1
FAtribut[2] := FAtribut[2] + 3
else if acFill in value then // byte 2, bit 0
FAtribut[2] := FAtribut[2] + 4;
end;procedure TXLSWriter.WriteWord(w: word);
begin
fstream.Write(w, 2);
end;procedure TXLSWriter.WriteEOF;
begin
WriteWord(BIFF_EOF);
WriteWord(0);
end;procedure TXLSWriter.WriteField(vCol, vRow: word; Field: TField);
begin
case Field.DataType of
ftString, ftWideString, ftBoolean, ftDate, ftDateTime, ftTime, ftWideMemo:
CellStr(vCol, vRow, Field.asstring);
ftAutoInc, ftSmallint, ftInteger, ftWord:
CellWord(vCol, vRow, Field.AsInteger);
ftFloat, ftBCD:
CellDouble(vCol, vRow, Field.AsFloat);
else
CellStr(vCol, vRow, EmptyStr); // <2002-11-17> dllee ??????????
end;
end;procedure TXLSWriter.WriteCodePage;
begin
WriteWord($0042); // OPCODE CODEPAGE
WriteWord($0002); // size
WriteWord($04E2); // CP1250
//- >http://sc.openoffice.org/excelfileformat.pdf , section 5.17
end;end.
-
On 12/23/2019 at 2:45 PM, lfgarrido said:
Hello, I'm looking for a way to export a UniDbGrid or some DataSet to Excel withou OLE approaches, because the server will not have Excel installed.
Also, I saw at 2019 roadmap an item "ExportableGrids"
Does anyone know if it's ready or have suggestions on how I can do without third party components?
TksI have a solution ... if you still need it, let me know when I post the code.
-
On 1/14/2016 at 7:59 AM, Sherzod said:
Hi,
Sorry, now it's clear,,
For now try, can help:
UniMemo1 -> ClientEvents -> ExtEvents ... add keydown fn:
function keydown(sender, e, eOpts) { if (e.getKey() == e.ENTER) { e.stopPropagation(); } }
Best regards.
Did not work ... Can you help me??
-
The problem was solved? I still have problem with locate after opening Dataset ...
-
Any prevision???
-
Good afternoon...
I have the following problem ...
User opens system with non-maximized browser screen.
After opening the screen he wants to work on, he decides to maximize the screen, but the unigui screen does not replay itself ...
Any suggestion?
Thanks...
Att.:
Gustavo Déo
-
Any prevision?
-
On 7/9/2018 at 4:12 PM, Ron said:
To access a local printer directly you have to set up and
go through a local process, and there are several ways to do that.
One is cross-origin resource sharing, CORS, and then the local
process runs an http server being triggered by a JS client call.
Another simple way is polling, like your local process does a
regular db polling to see if there is something to be printed.
A third way is to start messing with some addons like flash
or java to get direct hardware access. In this case the local
process is loaded on demand, in the previous cases it must
be running beforehand to serve the request.
Have an example using the flash plugin ???
-
On 5/14/2019 at 9:42 PM, Hayri ASLAN said:
It is not in the next 2 sprint. Most likely after that.
This sprint could be a priority ... It will help a lot in the speed of opening of screens ode there medium and large volume of data ... Any prediction?
-
1
-
-
-
On 4/17/2019 at 8:17 AM, Hayri ASLAN said:
It is our in backlog. We will implement this feature.
Any predictions for this implementation?
-
On 4/18/2019 at 9:37 AM, dpfmp said:
Hi,
In many grids, I use cell hints to display different information than the one that appears inside the cell.
For example, one cell contains information about a license number, the hint would display the client name that is associated with this license number.
I have a function in MainForm that returns the client firstName and lastName for that particular license:
function TMainForm.GetClientName(const Licence: integer): string;
var
S: string;
begin
with ClientTB do
begin
if FindKey([Licence]) then
Result := S + Trim(Fields[2].AsString) + ' ' + Trim(Fields[1].AsString);
else
Result := '';
end;
end;What I am trying to achieve is this:
I am using this code from here:
UniDbGrid.ClientEvents.ExtEvents
Finally my question is:
How can I access MainForm.GetClientName with record.data[3] as parameter so that I can display the information about the client in the tooltip?
Thank you,
Denis Prince
D10.1 UniGUI 1.70.0.1493
I was interested in this event ...
to simply show the content of the field ...
How do I make an event call when positioning the mouse without a grid?
Thank you !!!
Att.:
Gustavo Déo
-
Would you like to know more ... You can work with Fetch on Demand?
Thanks..
Att.:
Gustavo Déo..
-
Good night!!! Would there be a place to keep track of what's new in each release version of unigui?
Att.:
Gustavo Déo
-
35 minutes ago, Hayri ASLAN said:
Hi,
please use non blocking dialog. "MessageDlgN"
ohh... Perfect!!!
Thanks!!!
-
Good Morning!!! I am developing an application with using EnabledsynchronousOperation = True ... But when a code with treatment, as exemplified below ... try if not true then abort; except on e: exception MessageDlg (e.Message, mtError, [mbOk]); end; The System raises an internal exception, causing a PopUp message in the browser, and then raises the error message programmed in the unigui by missing the actual e.message error.
I did the test using EnabledsynchronousOperation = False and I did not have the same problem,
however I can not work that way due to the system architecture.
UniGui version 1.50
-
On 12/29/2018 at 7:09 PM, TI.ME said:
Good afternoon!!! http://forums.unigui.com/index.php?/topic/3275-messageserver-push-messages-from-server-to-client-long-polling/ Personally, I have adapted the message server into my project and it works perfect ... However, I moved the installation of my project to HyperServer, and now it does not work !!! When you start the msg screen, it javascript error msg !!! Has anyone ever had this problem ?? Would it help to solve ??
Error Msg
<script type="text/javascript">var w=window;var dc=w.document;w.onbeforeunload=null;dc.open();dc.write("\x3Chtml\x3E\n\x3Cbody bgcolor=\"#dfe8f6\"\x3E\n\x3Cp style=\"text-align:center;color:#0000A0\"\x3EInvalid session or session Timeout.\x3C/p\x3E\n\x3Cp style=\"text-align:center;color:#A05050\"\x3E\x3Ca href=\"http://localhost:8077/\"\x3ERestart application\x3C/a\x3E\x3C/p\x3E\n\x3C/body\x3E\n\x3C/html\x3E\n");dc.close();</script>
Good night... Actually it was problem with the port, with the hyperserver, the unigui executable, it does not use the default port configured, the hyperserver determines another port ... I made the correction and everything went back to normal !! Thank you!!!
-
1
-
-
Good afternoon!!! http://forums.unigui.com/index.php?/topic/3275-messageserver-push-messages-from-server-to-client-long-polling/ Personally, I have adapted the message server into my project and it works perfect ... However, I moved the installation of my project to HyperServer, and now it does not work !!! When you start the msg screen, it javascript error msg !!! Has anyone ever had this problem ?? Would it help to solve ??
Error Msg
<script type="text/javascript">var w=window;var dc=w.document;w.onbeforeunload=null;dc.open();dc.write("\x3Chtml\x3E\n\x3Cbody bgcolor=\"#dfe8f6\"\x3E\n\x3Cp style=\"text-align:center;color:#0000A0\"\x3EInvalid session or session Timeout.\x3C/p\x3E\n\x3Cp style=\"text-align:center;color:#A05050\"\x3E\x3Ca href=\"http://localhost:8077/\"\x3ERestart application\x3C/a\x3E\x3C/p\x3E\n\x3C/body\x3E\n\x3C/html\x3E\n");dc.close();</script>
-
On 1/26/2018 at 5:53 PM, jahlxx said:
Hi.
I'm testing new beta version with ext 6.5
I have 2 quiestions.
- This code don't work. I have it in mainform script:
Ext.override(Ext.view.Table, {
walkCells: function (pos, direction, e, preventWrap, verifierFn, scope) {
var grid = pos.view.headerCt.grid;
if ((direction == 'right' && grid.columnManager.columns[pos.column+1] ) ||
(direction == 'left' && grid.columnManager.columns[pos.column-1] ) ||
(direction == 'down' || direction == 'up'))
{
return this.callParent(arguments);
} else {
return false;
}
}
});
- This, in custom css, only show horizontal lines:
.x-grid-with-row-lines .x-grid-td {
border-color: black;
}
.x-grid-with-col-lines .x-grid-cell {
border-color: black;
}
- And this has a extrange behaviour too:
if (key = VK_NEXT) then
(sender as tunidbgrid).datasource.Dataset.RecNo := (sender as tunidbgrid).datasource.Dataset.RecNo + (sender as tunidbgrid).WebOptions.PageSize;if (key = VK_PRIOR) then
(sender as tunidbgrid).datasource.Dataset.RecNo := (sender as tunidbgrid).datasource.Dataset.RecNo - (sender as tunidbgrid).WebOptions.PageSize;if (Key = VK_DOWN)and((Sender as TUniDBGrid).WebOptions.Paged) then begin
if ((Sender as TUniDBGrid).DataSource.DataSet.RecNo mod (Sender as TUniDBGrid).WebOptions.PageSize) = 0 then
(Sender as TUniDBGrid).DataSource.DataSet.Next
end;
if (Key = VK_UP)and((Sender as TUniDBGrid).WebOptions.Paged) then begin
if (((Sender as TUniDBGrid).DataSource.DataSet.RecNo - 1) mod (Sender as TUniDBGrid).WebOptions.PageSize) = 0 then
(Sender as TUniDBGrid).DataSource.DataSet.Prior
end;
Thanks.
Your code was useful ...
I just improved a bit to avoid mistakes and stay clean !!!
I hope it helps you tb ...But I only have one difficulty ... if I hold the key pressed, debugging the code, to realize that there is a very large delay ... so sometimes the strange behavior that you quoted ..
But it's better than nothing !!!
Thank you friend!!!
procedure TfCadastro.grdCadastroKeyDown(Sender: TObject; var Key: Word;
Shift: TShiftState);
beginif (Sender as TUniDBGrid).WebOptions.Paged then
begin
case key of
VK_NEXT : begin
if (((sender as tunidbgrid).datasource.Dataset.RecNo + (sender as tunidbgrid).WebOptions.PageSize) > (sender as tunidbgrid).datasource.Dataset.RecordCount) then
(sender as tunidbgrid).datasource.Dataset.Last
else
(sender as tunidbgrid).datasource.Dataset.RecNo := (sender as tunidbgrid).datasource.Dataset.RecNo + (sender as tunidbgrid).WebOptions.PageSize;
end;VK_PRIOR : begin
if (((sender as tunidbgrid).datasource.Dataset.RecNo - (sender as tunidbgrid).WebOptions.PageSize) < 1) then
(sender as tunidbgrid).datasource.Dataset.First
else
(sender as tunidbgrid).datasource.Dataset.RecNo := (sender as tunidbgrid).datasource.Dataset.RecNo - (sender as tunidbgrid).WebOptions.PageSize;
end;VK_DOWN : begin
if ((Sender as TUniDBGrid).CurrRow = ((Sender as TUniDBGrid).WebOptions.PageSize) -1) then
(Sender as TUniDBGrid).DataSource.DataSet.Next;
end;
VK_UP : begin
if ((Sender as TUniDBGrid).CurrRow = 0) then
(Sender as TUniDBGrid).DataSource.DataSet.Prior;
end;end;
end;end;
-
On 10/26/2018 at 3:51 AM, Sherzod said:
Hello,
Maybe as a "workaround", try:
1. "pagingBar":
function pagingBar.beforechange(sender, page, eOpts) { sender.isAfterScroll=false; } function pagingBar.change(sender, pageData, eOpts) { Ext.defer(function() { sender.isAfterScroll=true; }, 200); }
2. "panel":
function afterrender(sender, eOpts) { var me = sender; me.view.getEl().on('scroll', function(e, t) { if (me.pagingBar.isAfterScroll) { if (t.scrollHeight - (t.scrollTop + me.view.el.getHeight()) == 0) { if (me.pagingBar) { me.pagingBar.moveNext(); } } else if (t.scrollTop == 0) { if (me.pagingBar) { me.pagingBar.movePrevious(); } } } }); }
For the previous page, it worked ... But it did not work for the next page.
HyperServer + IIS - ON line (Work) and LocalHost (not work).
in General
Posted
Good afternoon,
I published the system using the hyperserver + IIS for external access, it is working perfectly. But I can't access via localhost. Can someone help me?
Thanks.